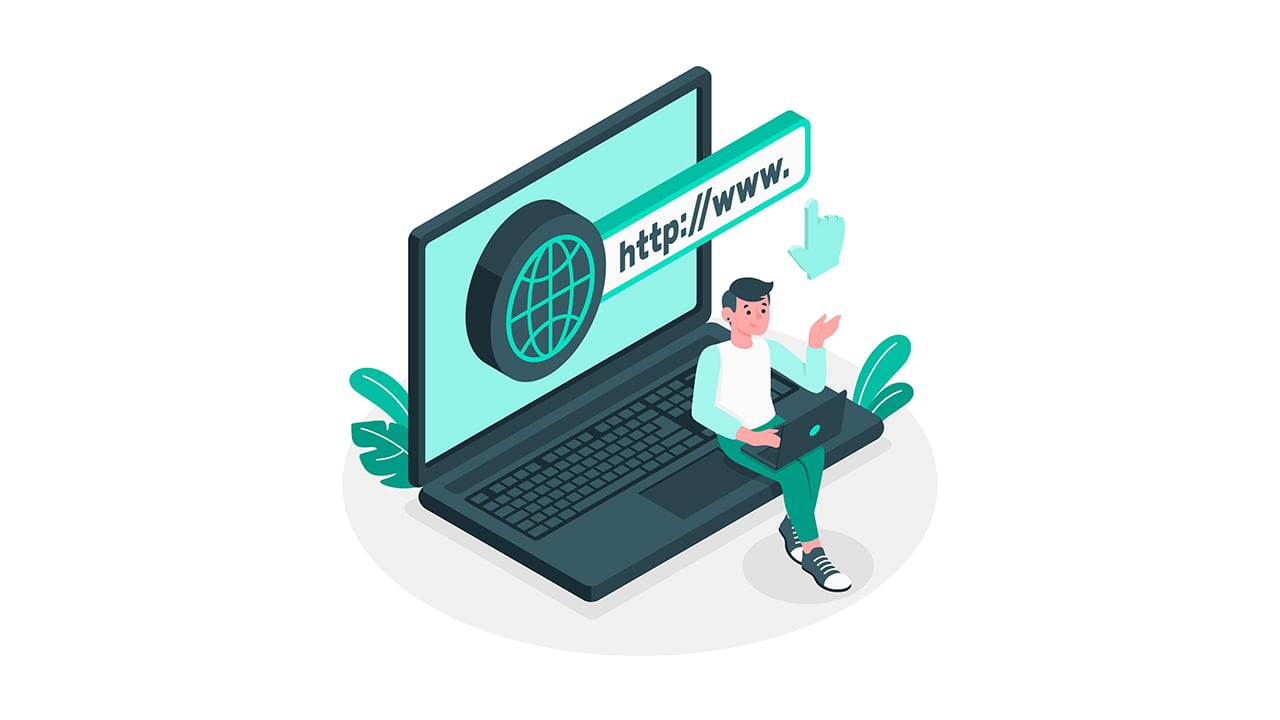
4 minutes read
How to redirect to another page in PHP
Table of contents
- → Introduction
- → Quick PHP redirect example (TL;DR)
- → HTTP headers explained
- → Absolute vs. relative URLs
- → Choosing the correct HTTP status code
- → Alternative redirect methods (meta refresh & JavaScript)
- → SEO considerations: redirect chains and caching
- → Best practices
- → Clearer PHP redirection with http_response_code()
- → Verifying redirects
Introduction
Performing a redirect in PHP is straightforward, but mastering redirects involves understanding HTTP status codes, avoiding common pitfalls, keeping SEO considerations in mind, and ensuring security best practices.
Quick PHP redirect example (TL;DR)
Here’s the essential PHP redirect code snippet:
// Start output buffering to avoid "headers already sent" errors. ob_start(); header('Location: https://example.com', true, 301); // Permanent redirect. exit;
Let’s break down what’s happening here:
- Output buffering (
ob_start()
): Ensures nothing is sent to the browser prematurely, preventing PHP “headers already sent” errors. header()
function: Directs browsers to the specified URL.- HTTP status code (301): Indicates this redirect is permanent, beneficial for SEO.
exit
: Stops PHP execution immediately after redirection.
HTTP headers explained
When you redirect with PHP, the server sends an HTTP response like this:
HTTP/1.1 301 Moved Permanently Location: https://example.com Content-Type: text/html
The important elements:
- Status code (301): Signals browsers and search engines this URL has moved permanently.
- Location header: Indicates the new URL destination.
Avoid echoing text before sending headers. Output buffering (ob_start()
) reliably prevents “headers already sent” errors.
Absolute vs. relative URLs
Always use absolute URLs (https://example.com/page
) in PHP redirects. Relative URLs (/page
) may fail with proxies or older browsers, causing unpredictable behavior.
Choosing the correct HTTP status code
PHP defaults to a 302 Found
redirect if no status code is specified:
header('Location: https://example.com'); // defaults to 302
Explicitly choose the correct status code for SEO:
Status | Meaning | Method preserved? | Cache behavior |
---|---|---|---|
301 | Permanent redirect | No | Aggressively cached |
302 | Temporary redirect | No | Rarely cached |
303 | Redirect after form submission (PRG) | No | Not cached |
307 | Temporary redirect | Yes | Temporarily cached |
308 | Permanent redirect | Yes | Aggressively cached |
Example:
// Temporary redirect, preserves POST method. header('Location: https://example.com/temp-page', true, 307); exit;
HTTP 308 permanent redirect
The 308 status code preserves the request method (e.g., POST), making it ideal for migrating APIs or services where method consistency matters.
header('Location: https://api.example.com/new-endpoint', true, 308); exit;
Alternative redirect methods (meta refresh & JavaScript)
Use server-side PHP redirects whenever possible. Meta refresh and JavaScript redirects should only be fallback solutions:
Meta refresh
Meta refresh redirects occur within HTML tags. Immediate refreshes (content="0"
) act as permanent redirects; delayed refreshes (content="5"
) are treated as temporary. Example:
<meta http-equiv="refresh" content="0;url=https://example.com">
JavaScript redirects
JavaScript redirects rely on client-side execution and might not be reliable for all users. They’re often slower and can negatively affect SEO. Example:
window.location.href = 'https://example.com';
SEO considerations: redirect chains and caching
Googlebot follows a maximum of 10 redirect hops. Long chains negatively impact SEO and performance. Consolidate redirect chains whenever possible.
Permanent redirects (301
, 308
) are aggressively cached by browsers and CDNs. For anticipated changes or A/B testing, use temporary codes (302
, 307
).
Each redirect adds latency, affecting Core Web Vitals, critical to Google’s ranking signals in 2025. Optimize redirects to minimize delays.
Best practices
- Prefer server-level redirects (e.g.,
.htaccess
, Nginx). - Avoid redirect chains and loops.
- Regularly update internal links instead of relying on redirects.
- Monitor redirects with tools like Google Search Console or Screaming Frog.
Security: avoiding open redirects
Never directly redirect to URLs from user input without validation, risking phishing attacks.
Secure example with URL whitelist:
$allowed_urls = ['/dashboard', '/profile', '/home']; $next = $_GET['next'] ?? '/home'; if (!in_array($next, $allowed_urls, true)) { $next = '/home'; } header("Location: $next", true, 303); exit;
Clearer PHP redirection with http_response_code()
Explicitly set HTTP status codes for clarity:
ob_start(); http_response_code(301); header('Location: https://example.com'); exit;
Verifying redirects
Use curl
to verify redirects from the command line:
curl -I https://your-site.com/old-page
Expected output:
HTTP/1.1 301 Moved Permanently Location: https://your-site.com/new-page
Browser developer tools also show redirect details clearly.
Did you like this article? Then, keep learning:
- Explore PHP 8.4's new features for up-to-date coding practices
- Know how to find out your PHP version quickly and easily
- Learn how to convert PHP arrays to JSON for APIs and data exchange
- Master handling PHP exceptions using try and catch blocks
- Master printing arrays in PHP for better debugging experiences
- Improve your code with PHP's null coalescing operator explained
- Learn different types of PHP redirections beyond basics
- Debug PHP code effectively by showing all errors plainly
- Understand and solve common PHP errors like $this in non-object context
0 comments