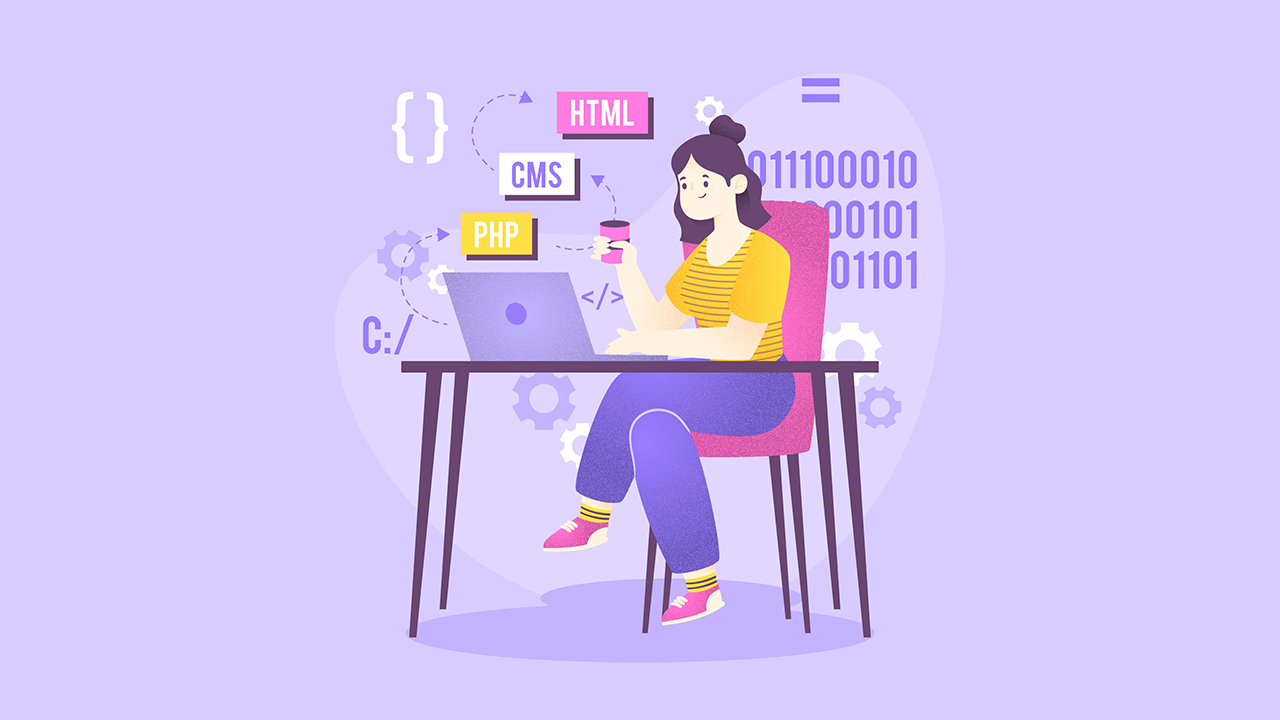
Print an array with PHP (+ Laravel)
Introduction to printing arrays in PHP
There are multiple ways to print the content of an array in PHP like var_dump()
, print_r()
, var_export()
, and even json_encode()
.
Let me review each of them in this article.
Print a PHP array using print_r()
print_r()
displays arrays in a human-readable format.
Example:
print_r(['Foo', 'Bar', 'Baz']);
Output:
Array ( [0] => Foo [1] => Bar [2] => Baz )
If you need to capture the output instead of echoing it, you can pass a second parameter to print_r()
:
$output = print_r(['Foo', 'Bar', 'Baz'], true);
Print a PHP array using var_dump()
var_dump()
prints information about any type of value. It works great for arrays too!
Example:
var_dump(['Foo', 'Bar', 'Baz']);
Output:
array(3) { [0]=> string(3) "Foo" [1]=> string(3) "Bar" [2]=> string(3) "Baz" }
You can also print an infinite number of variables at once:
var_dump($foo, $bar, $baz, …);
Print a PHP array using var_export()
var_export()
prints a parsable string representation of a variable that you could just copy and paste into your source code.
Example:
$array = ['Foo', 'Bar', 'Baz']; var_export($array);
Output:
array ( 0 => 'Foo', 1 => 'Bar', 2 => 'Baz', )
Print a PHP array using json_encode()
json_encode()
can print arrays as JSON.
Example:
$array = ['Foo', 'Bar', 'Baz']; echo json_encode($array);
Output:
["Foo","Bar","Baz"]
Print a PHP array using Laravel’s dump() function
{: width=“400”}
The dump()
function prints in detail arrays containing any value.
$array = ['Foo', 'Bar', 'Baz']; dump($array);
And just like var_dump()
, it accepts an infinite number of arguments:
dump($a, $b, $c, $d, $e, …);
Print a PHP array using Laravel’s dd() function
The dd()
function does the same thing as dump()
, but stops code execution.
$array = ['Foo', 'Bar', 'Baz']; dd($array);
It also accepts an infinite number of arguments:
dd($a, $b, $c, $d, $e, …);
Did you like this article? Then, keep learning:
- Discover various methods to check your PHP version effectively for troubleshooting
- Learn how to clear Laravel's cache to resolve various common issues easily
- Dive deeper into PHP debugging especially with Laravel's dump() and dd() functions
- Learn how to handle and fix a common PHP error with foreach loops
- Learn best practices to validate array data in Laravel forms and APIs with ease
- Explore Laravel's Collections to handle arrays more powerfully and conveniently
- Understand Laravel's maintenance mode to safely manage your application's downtime
- Understand and fix common Laravel application encryption key errors quickly
- Master Laravel's query builder and its powerful where clauses
- Learn efficient filtering of database queries in Laravel using whereIn()