Here's the fix to "using $this when not in object context."
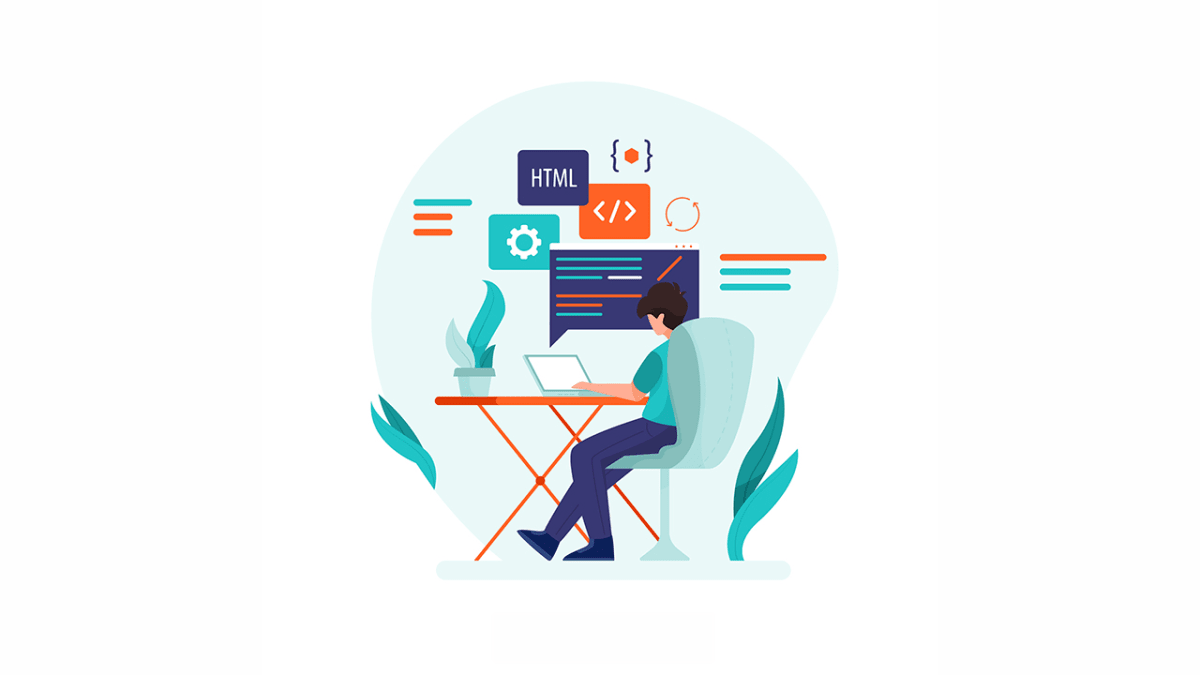
Introduction
To fix “Using $this when not in object context”, you can make the static method that is calling $this
non-static.
No matter if you’re using CodeIgniter, CakePHP, Laravel, Symfony, WordPress, Yii, or anything else, $this
is a variable that refers to the current object. Therefore, it’s natural to not being allowed to call it from a static method.
How to fix “Using $this when not in object context”, by example
Take this code and try to run it. You will see “Using $this when not in object context” again.
class Foo { public static function bar() { // This is bad because we are in a static method. $this->baz(); } public function baz() { } } Foo::bar();
As you can see, we are trying to call baz()
, which is a non-static method, from a static method.
As mentioned above, we need to:
- Remove the
static
keyword frombar()
’s declaration; - Create an instance of
Foo
and callbar()
from there.
class Foo { public function bar() { $this->baz(); } public function baz() { } } $foo = new Foo; $foo->bar();
You could also make the baz method static depending on your initial intention:
class Foo { public static function bar() { static::baz(); } public static function baz() { } }