PHP's double question mark, or the null coalescing operator
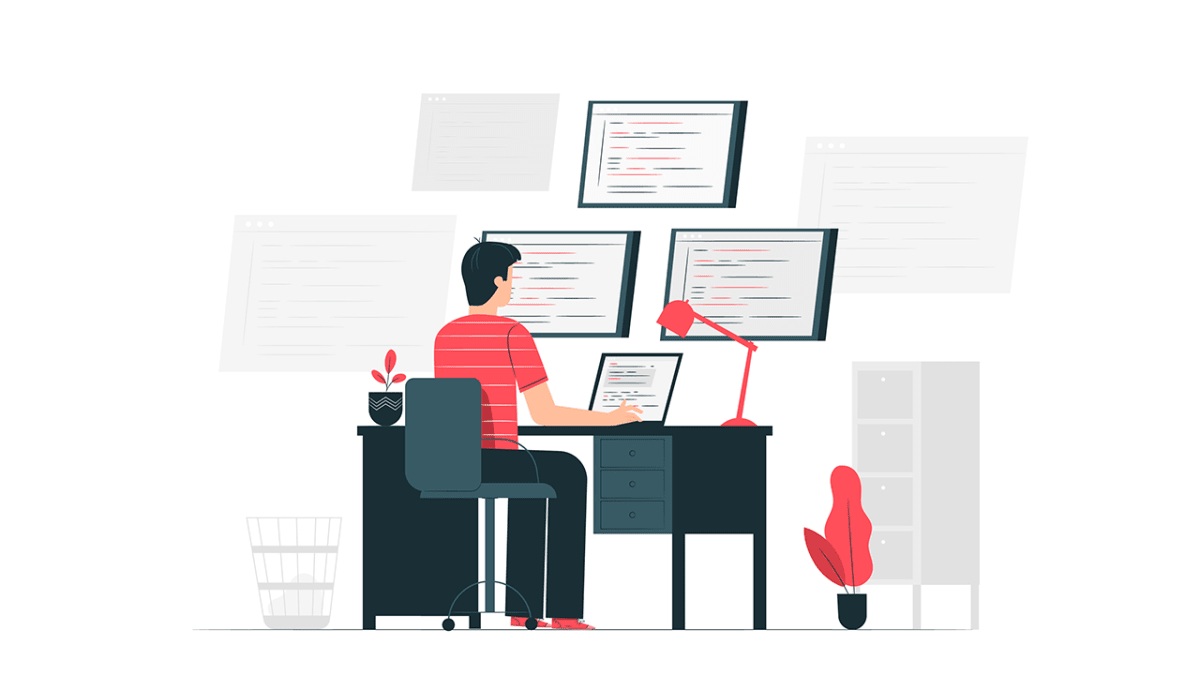
The null coalescing operator (??), or the double question mark
The null coalescing operator, or double question mark, was introduced in PHP 7.0 and is a handy shortcut that helps you write cleaner and more readable code. It’s represented by two question marks ??
.
Let’s say you want to get a value from a variable, but if that variable is not set or is null
, you want to use a default value instead. You can use the ??
operator to do this in one step.
For example:
$name = $_GET['name'] ?? 'Unknown';
This line of code will set $name
to $_GET['name']
if it’s set and not null. Otherwise, it will set $name
to “Unknown”.
You can also chain them together like this:
$foo = $foo ?? $bar ?? 'baz';
This will check $foo
first, then $bar
, and use “baz” if neither are set and not null.
The null coalescing assignment operator (??=), or the double question mark equals
PHP 7.4 introduced a new shortcut, ??=
(double question mark equals), also called the null coalescing assignment operator. This is used when you want to set a variable to a new value only if it’s currently not set or null.
It’s hard to make up a good example, but here’s a simplified one from this very blog’s codebase:
function do_something(DateTime $from, DateTime $to = null) { // Using the ternary operator. $to = $to ? $to : new DateTime('now'); // Using the Elvis operator. $to = $to ?: new DateTime('now'); // Using the null coalescing assignment operator. $to ??= new DateTime('now'); // Do something. }
This will set $to
to a new DateTime
instance only if it’s not already set (or null
in that case).