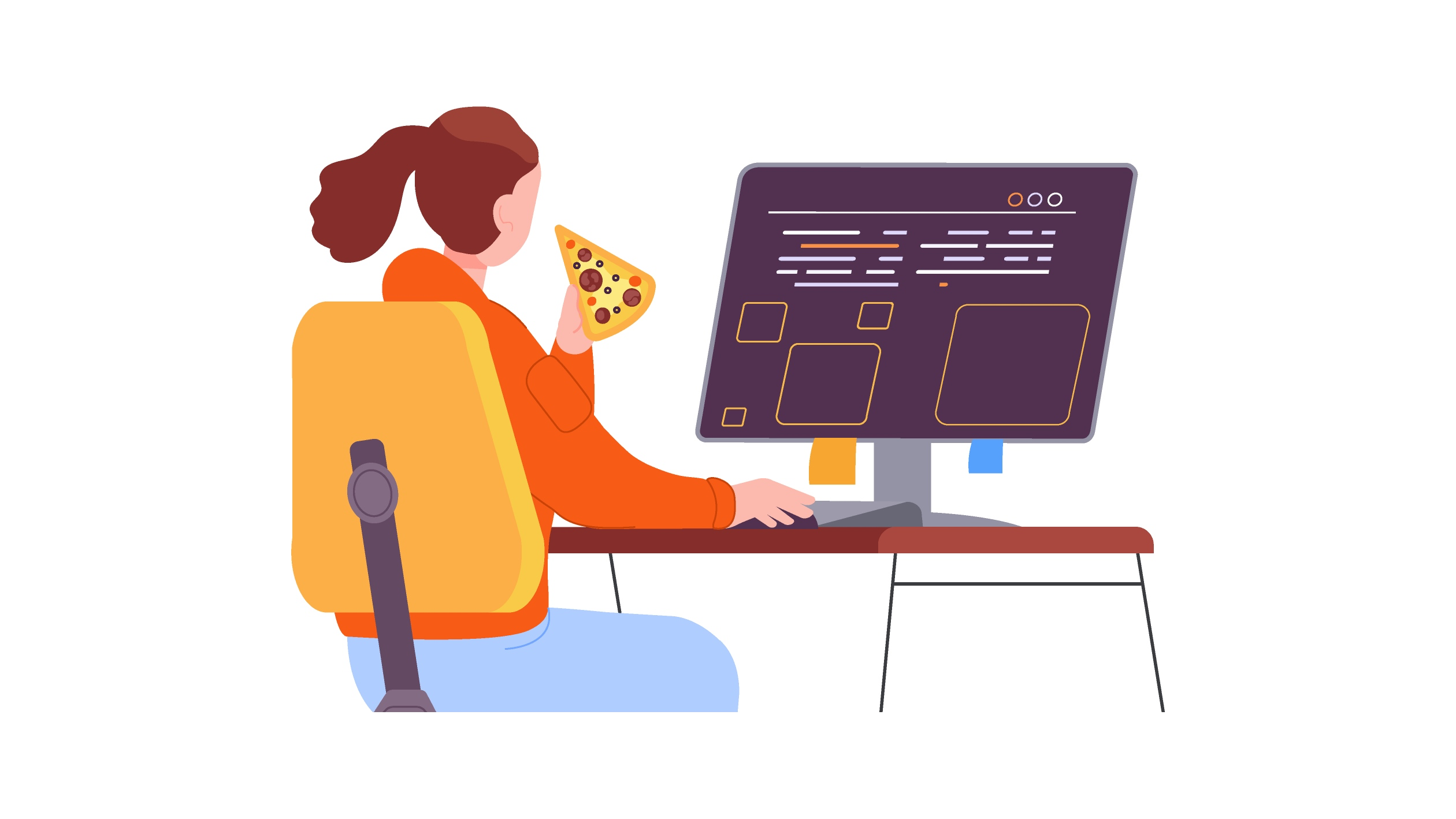
PHP's str_replace() made simple
Introduction
PHP provides a plethora of built-in functions, with str_replace()
being one of my favorites.
This function is fundamental to handling and manipulating strings, playing an essential role in many PHP applications.
The basics of the str_replace() function in PHP
The str_replace()
function in PHP replaces strings with other strings. It’s that simple, yet powerful.
Its signature looks like this:
str_replace( string|array $search, string|array $replace, string|array $subject, int &$count = null ) : string|array
search
: It specifies the value to find. It can either be astring
or anarray
.replace
: It defines the value to replace the found value with. It can either be astring
or anarray
.subject
: It’s the string or array to be searched and replaced on. It can either be astring
or anarray
.count
: It’s an optionalinteger
and determines the number of replacements performed.
To me, examples speak more than theory. Let’s explore how to use str_replace()
.
Practical use cases of str_replace() in PHP
Classic search and replace
Let’s say we have a greeting “Hello, unknown person!” and we want to change “unknown person” to “Benjamin”.
Using str_replace()
, we can achieve this like so:
$sentence = 'Hello, unknown person!'; echo str_replace('unknown person', 'Benjamin', $sentence); // Outputs: Hello, Benjamin!
Search and replace multiple values
str_replace()
can also be used with arrays, sequentially searching and replacing the values.
This can avoid you having to call str_replace()
multiple times.
For example:
$sentence = '1st, 2nd, and 3rd.'; echo str_replace( ['1st', '2nd', '3rd'], ['first', 'second', 'third'], $sentence ); // Outputs: first, second, and third.
Search and replace in an array of strings
str_replace()
accepts a value of type array
as the subject.
Which means you can still avoid calling str_replace
multiple times if you are able to build an array of subjects. I didn’t know that before writing this article!
Let me show you:
$sentences = [ 'You cannot mention Mastodon on Twitter!', "Let's build a new society on Mastodon!", 'Is Mastodon a Twitter-killer?', ]; var_dump( str_replace( 'Mastodon', '@&$!?%', $sentences ) ); // Outputs: [ // 'You cannot mention @&$!?% on Twitter!', // "Let's build a new society on @&$!?%!", // 'Is @&$!?% a Twitter-killer?', // ]
PHP’s str_ireplace() isn’t case sensitive
The str_replace() function is case-sensitive.
If you need case-insensitive replacement, PHP offers the str_ireplace()
function.
echo str_ireplace('foo', 'bar', 'FOo foo fOO'); // Outputs: bar bar bar
The limitations of the str_replace() function in PHP
While versatile, str_replace()
has its limitations:
- No direct support for regular expressions: As mentioned,
str_replace()
does not support regex. For this, you’ll need to usepreg_replace()
. - It’s unable to replace multi-byte unicode characters: The
str_replace()
function is not safe for multi-byte characters like those found in UTF-8 strings. - There are problems with case sensitivity: As noted before,
str_replace()
is case-sensitive. If you need to ignore case, usestr_ireplace()
.
Did you like this article? Then, keep learning:
- Discover PHP 8.3 new Override attribute benefits for cleaner code
- Learn about PHP's upcoming major version and breaking changes
- Understand PHP array manipulation to complement string manipulation
- Master converting PHP arrays to JSON for API communication
- Explore PHP's array_map() for advanced array processing
- Learn efficient methods to sort any kind of PHP array
- Handle PHP exceptions elegantly with try & catch blocks
- Understand how to print arrays for debugging in PHP and Laravel