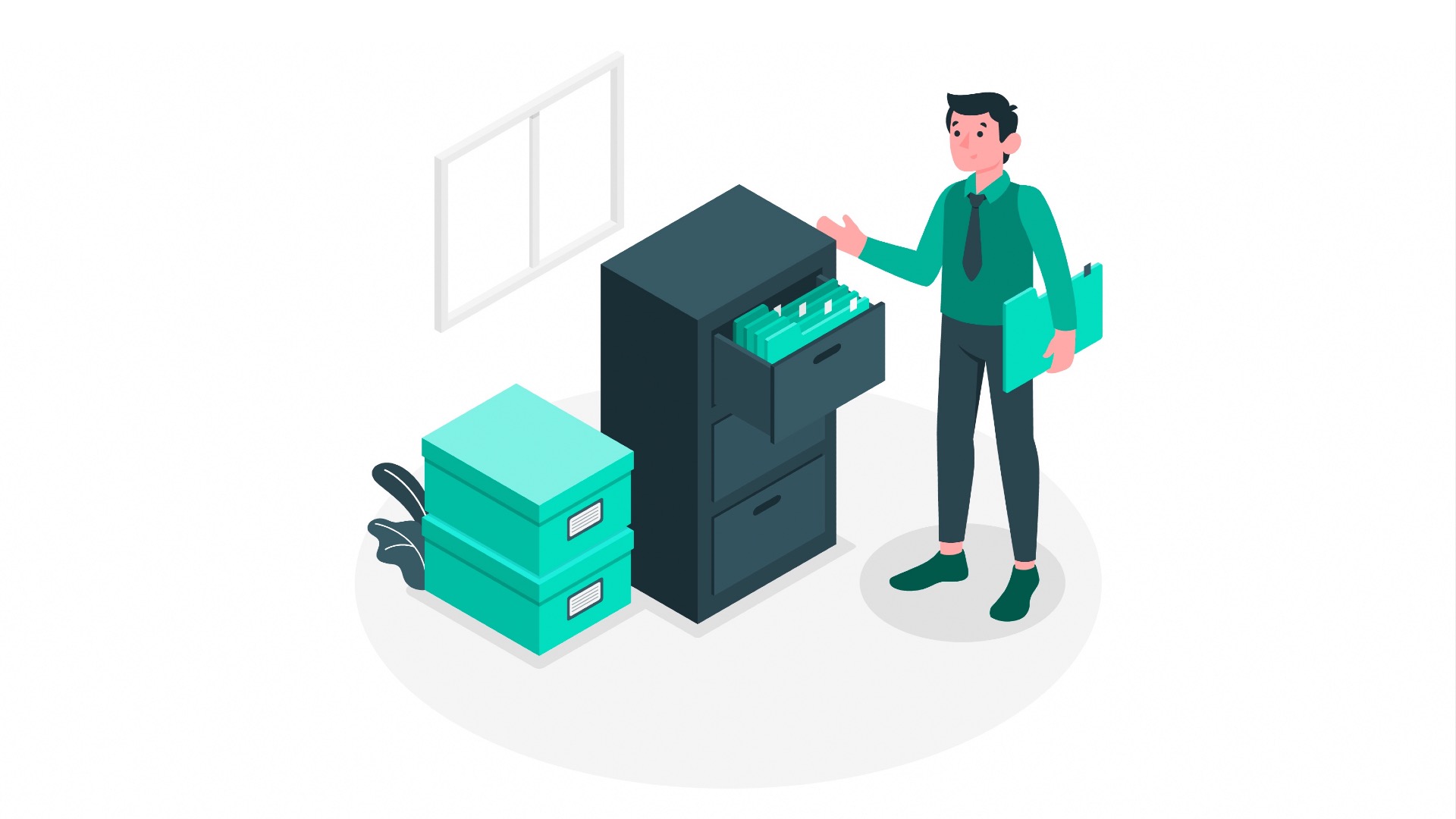
Learn how to sort any kind of array in PHP
Sorting arrays is a common task in PHP, and the language provides a variety of functions to order elements just the way you need.
Whether you’re dealing with numerical indices or associative arrays, PHP has you covered. 😎
Let me show you some of PHP’s array sorting capabilities.
Utilizing the sort() and rsort() Functions
When you have a simple indexed array that needs reordering, sort()
and rsort()
are the straightforward choices for ascending and descending order, respectively.
For sort()
:
$fruits = ["Banana", "Apple", "Orange"]; sort($fruits); var_dump($fruits);
Once sorted, the $fruits
array will be:
["Apple", "Banana", "Orange"]
Now, what to do when you have an associative array? The sort()
function won’t cut it.
Maintaining key association with asort() and arsort()
Associative arrays require maintaining the relationship between keys and values. asort()
sorts in ascending order by value, maintaining key association, and arsort()
does the same in descending order.
Example of asort()
:
$prices = [ "Apple" => 1.2, "Banana" => 0.5, "Orange" => 0.9, ]; asort($prices); var_dump($prices);
The sorted $prices
array now looks like this:
[ "Banana" => 0.5, "Orange" => 0.9, "Apple" => 1.2, ]
Key-centric sorting with ksort() and krsort()
To sort an associative array by its keys, ksort()
for ascending order and krsort()
for descending order are your go-to functions.
Using ksort()
:
$products = [ "product3" => "Chair", "product1" => "Desk", "product2" => "Lamp", ]; ksort($products); var_dump($products);
Custom sorting with usort(), uasort(), and uksort()
What we saw until now will probably cover 99% of your needs.
But sometimes, you need a sorting logic that’s not built-in. For these instances, usort()
for value-based, uasort()
for value-based with preserved keys, and uksort()
for key-based custom sorting. Each requires a user-defined comparison function.
An example with usort()
:
$numbers = [3, 2, 5, 6, 1]; usort($numbers, function ($a, $b) { return $a <=> $b; // The spaceship operator }); var_dump($numbers);
With the custom function, $numbers
will be sorted as:
[1, 2, 3, 5, 6];
Of course, in that case, using sort()
would be better. I just wanted to let you know that custom sorting logic is possible! 🙂
Did you like this article? Then, keep learning:
- An easy guide to check PHP version to ensure compatibility before coding
- Unlock Laravel Collections tips to write cleaner, more efficient PHP array code
- Master Laravel Eloquent sorting with orderBy() for database query results
- Explore PHP 8.3 features to write better, modern PHP code with new syntax
- Discover how to check if your PHP array is empty in the fastest way
- Learn to filter array elements effectively with PHP's array_filter() function
- Learn to efficiently convert PHP arrays to JSON for data exchange
- Discover the powerful array_map() function to transform your PHP arrays
- See how to print arrays in PHP and Laravel for easy debugging
0 comments