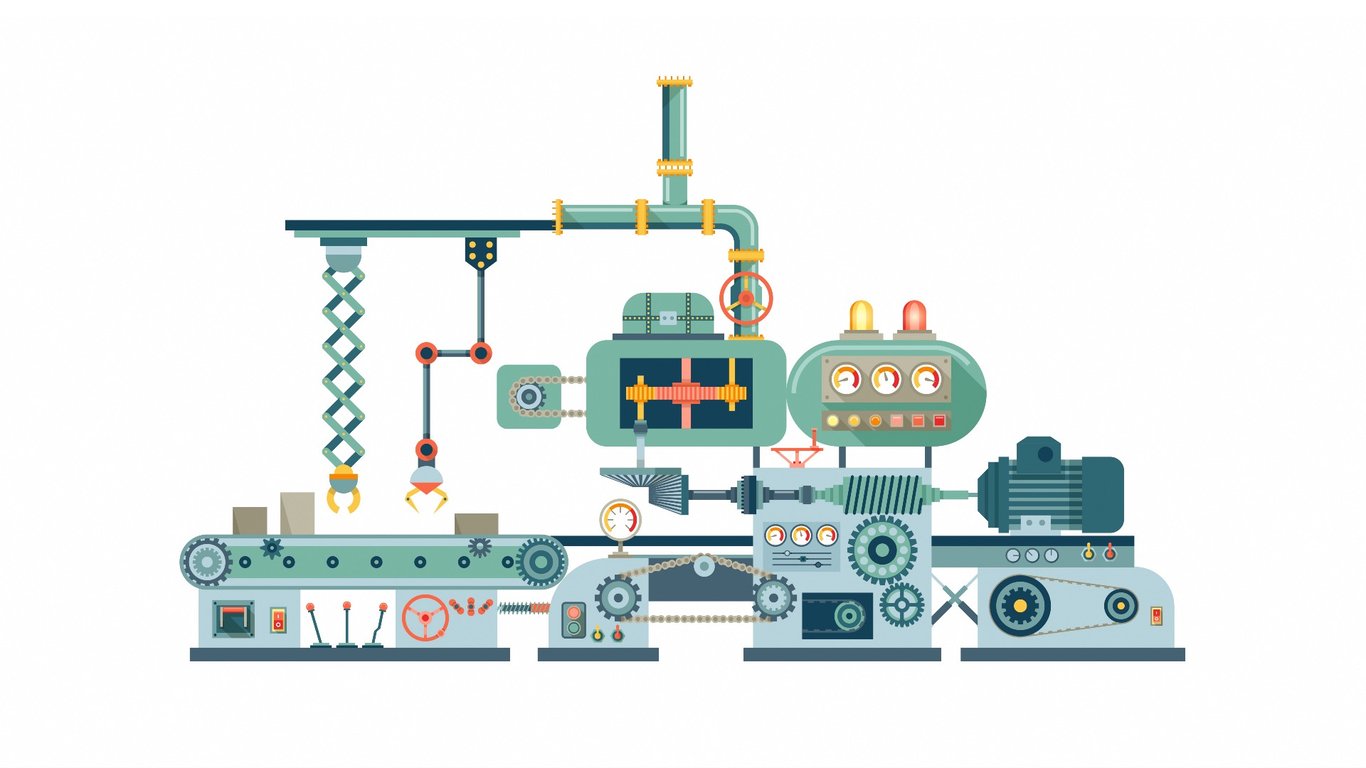
2 minutes read
Making sense of PHP's array_map() function
Table of contents
About array_map()
The array_map()
function in PHP is extremely useful to write leaner code when transforming arrays and avoid lengthier loops with temporary variables and an additional indentation level.
When I was inexperienced with PHP, I had a hard time understanding the array_map()
function. Now, things have changed, so let me desmystify this super handy function for you!
How to use array_map() in PHP
The array_map()
takes a callable as its first parameter, and the array we want to transform as its second parameter. It then returns the a fresh array that has freshly been transformed.
Now, imagine we have an array of prices that we want to apply a discount to.
Before array_map()
, we would use a code that looks like this:
function discount($price) { return $price * (1 - 0.2); } $prices = [ 10, 20, 30, 40, 50, ]; $discounted_prices = []; foreach ($prices as $price) { $discounted_prices[] = discount($price); }
This is good, but we can do better thanks to array_map()
:
function discount($price) { return $price * (1 - 0.2); } $prices = [ 10, 20, 30, 40, 50, ]; $discounted_prices = array_map(discount(...), $prices);
$discounted_prices
now contains:
[ 8, 16, 24, 32, 40, ];
Also, did you know that array_map()
can also accept a closure (or anonymous function)?
$prices = [ 10, 20, 30, 40, 50, ]; $discounted_prices = array_map(function ($price) { return $price * (1 - 0.2); }, $prices);
But wait, did you really think this is it? Why not use the arrow syntax on our closure?
$prices = [ 10, 20, 30, 40, 50, ]; $discounted_prices = array_map( fn ($price) => $price * (1 - 0.2), $prices );
There you have it! Using array_map()
can drastically help improving your code. Now, you can take a look at other similar functions such as array_filter()
or array_reduce()
.
One more thing about array_map()
Ha! You thought that was really it, right? No! array_map()
is such a useful function to use in PHP!
So, what you probably didn’t know is that it can accept an infinite amount of arrays to loop through. Here’s an example:
$products = [ 'Apple Watch', 'iMac', 'iPhone', 'Mac Studio', 'MacBook Pro', ]; $prices = [ 10, 20, 30, 40, 50, ]; $discounted_products = array_map(function ($product, $price) { return [ 'product' => $product, 'discounted_price' => $price * (1 - 0.2) ]; }, $products, $prices);
In this block of code, we build a unique multidimensional array out of two dinstinct ones and apply the discount.
[ [ 'product' => 'Apple Watch', 'discounted_price' => 8, ], … ]
Did you like this article? Then, keep learning:
- Discover Laravel Collections tips which offer advanced array manipulation capabilities
- Better understand Laravel's query building, useful if transforming data via PHP arrays
- Master checking for empty arrays efficiently, a common requirement after array operations
- Explore PHP's array_filter() to complement array_map() for array transformations
- Discover how to convert PHP arrays to JSON for API communication
- Learn PHP array sorting techniques to organize data effectively
- Simplify array value re-indexing with array_values(), useful after mapping
- Get guidance on catching and handling exceptions to improve PHP error handling
- Learn to debug PHP by printing arrays, enhancing troubleshooting skills
0 comments