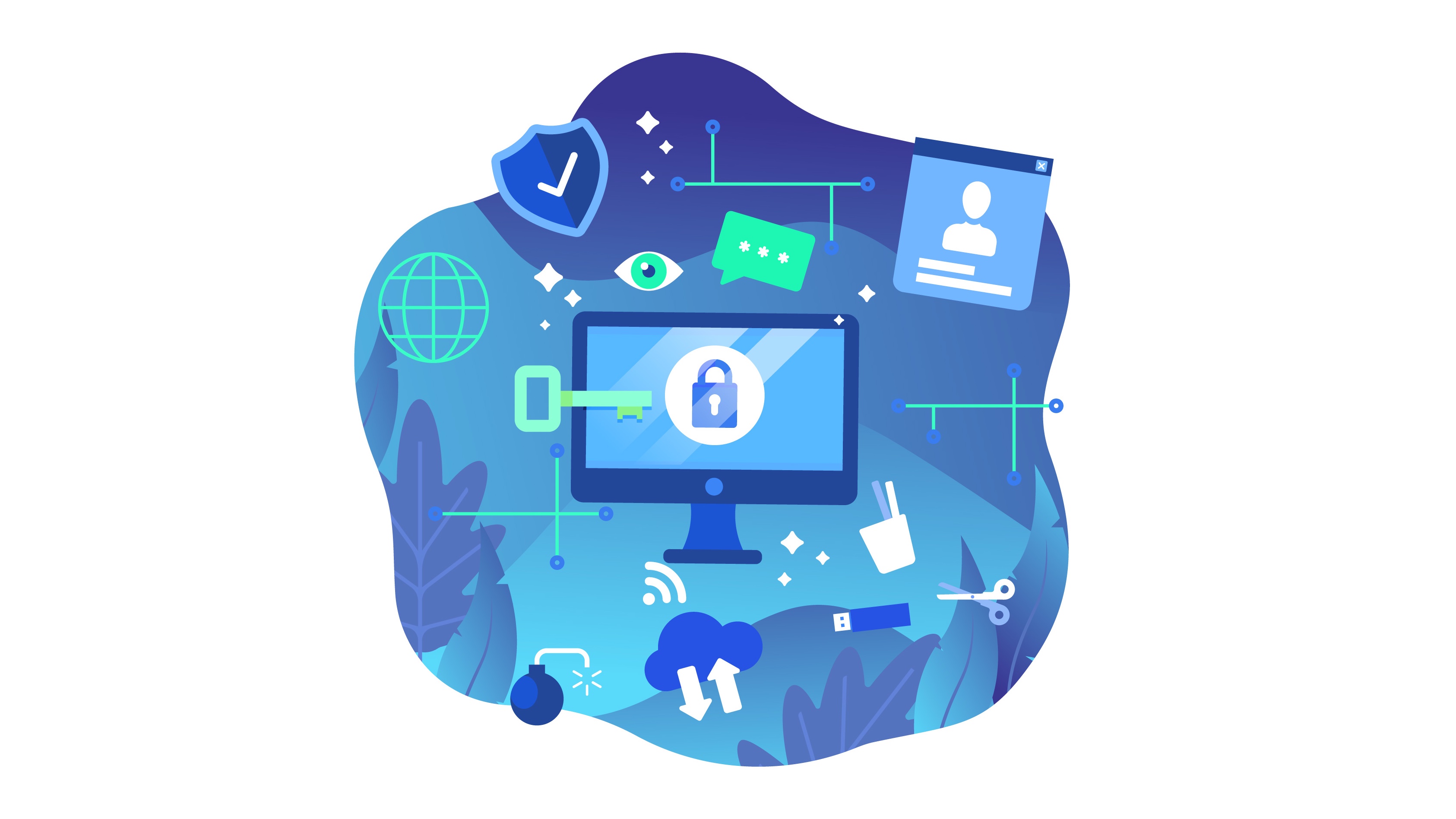
2 minutes read
Secure your REST API in 5 minutes with Laravel Sanctum
Table of contents
Introduction to Laravel Sanctum and how it helps securing REST APIs
Laravel Sanctum is a package for Laravel that provides a simple way to secure your REST API. For instance, in case you want your users to be able to build services top of your application.
That being said, the official documentation is extensive and you probably don’t have that kind of time. So I hope my quick guide will serve you well.
Install Laravel Sanctum via Composer
The package now comes installed by default in any new Laravel application.
If for some reason you don’t have Laravel Sanctum in your project, install it using Composer:
composer require laravel/sanctum
Once done, publish Sanctum’s configuration and migration files:
php artisan vendor:publish --provider="Laravel\Sanctum\SanctumServiceProvider"
Finally, run your database migrations:
php artisan migrate
Issue API tokens to your users
You need to let your users generate tokens to consume your API.
Add the Laravel\Sanctum\HasApiTokens
trait in your User
model:
namespace App\Models; use Laravel\Sanctum\HasApiTokens; // [tl! ++] class User extends Authenticatable { use HasApiTokens; // [tl! ++] }
You can issue a token using the createToken
method:
$token = $user->createToken('token-name')->plainTextToken;
Make sure to let the user know that the token is only shown once. If they lose it, they’ll have to generate a new one.
Protect your REST API routes with Sanctum’s auth guard
To secure your API routes, use the sanctum
guard. This ensures that all incoming requests are authenticated:
Route::middleware('auth:sanctum') ->get('/api/user', function (Request $request) { return $request->user(); });
Manage your users’ API tokens
Managing tokens is crucial for security. To revoke them, use:
// Revoke all tokens. $user->tokens()->delete(); // Revoke a specific token. $user->tokens()->where('id', $tokenId)->delete();
Conclusion
Securing your REST API with Laravel Sanctum is an effective way to manage authentication and prevent misuses without overcomplicating everything.
There’s a lot more to Laravel Sanctum and I encourage you to go read the official documentation.
Did you like this article? Then, keep learning:
- Learn how to manage Laravel cache effectively after securing APIs
- Discover effective error handling in Laravel HTTP client useful for APIs
- Introductory guide to Vanilla Laravel features that complement Sanctum usage
- Upgrade guide for latest Laravel versions to keep your API modern and secure
- Best practices specifically for building RESTful APIs with Laravel
- Expand your knowledge on securing Laravel APIs with broader security practices
- Learn to handle Laravel authentication UI scaffolding quickly for APIs
- Understand Laravel's Artisan CLI which helps in API and token management
0 comments