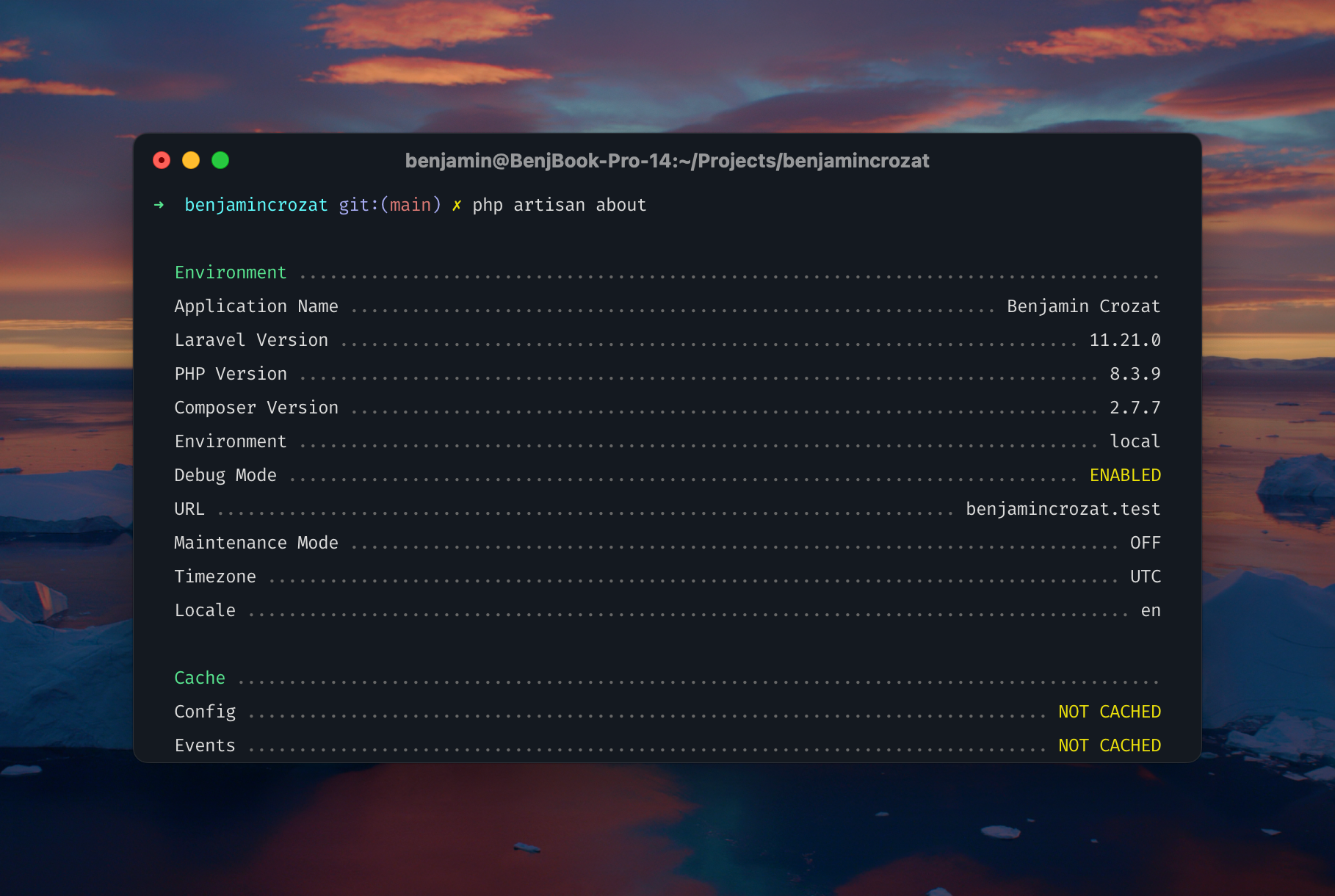
Demystifying Artisan: Laravel's magical command tool
- → Introduction
- → Getting Started with Artisan
- → Common Artisan Command Options
- → Artisan Commands: A Comprehensive Guide
- → Creating Custom Artisan Commands
- → Artisan Command Tips and Tricks
- → Conclusion
Introduction
What is Artisan?
Artisan is the command-line interface included with Laravel.Think of Artisan as your go-to helper for all sorts of tasks, from setting up databases to clearing out old stuff in your app. Every Laravel project comes with Artisan, ready to help you streamline your development process.
Why is Artisan crucial for Laravel developers?
I can’t stress enough how important Artisan is in the Laravel ecosystem. It’s not just a nice-to-have tool; it’s an essential part of Laravel development. Here’s why:
- Productivity Boost: Artisan automates many routine tasks, saving you time and reducing the chance of errors.
- Consistency: It ensures that certain operations are performed in a standardized way across your project.
- Extensibility: You can create your own custom Artisan commands to fit your project’s specific needs.
- Learning Tool: As you use Artisan, you’ll gain a deeper understanding of Laravel’s structure and best practices.
Getting Started with Artisan
How to access Artisan
Accessing Artisan is super easy. Open your terminal, navigate to your Laravel project’s root directory, and type:
php artisan
This command will display a list of all available Artisan commands. Running this command is like getting a cheat sheet for all the cool tricks Artisan can do. And that’s actually what I used to write this article!
Basic syntax and structure
The basic structure of an Artisan command is:
php artisan command:name {argument} {--option}
command:name
is the name of the command you want to run.represents required input.
{--option}
represents optional flags or parameters.
For example, to create a new controller, you might use:
php artisan make:controller UserController
Common Artisan Command Options
Before we dive into specific commands, let’s talk about some options that are available for almost every Artisan command. These are like the basic wand movements every wizard should know:
-h
or--help
: Shows help for the command. It’s like asking Artisan, “What does this spell do?”-q
or--quiet
: Suppresses all output. Useful when you’re running commands in scripts.-V
or--version
: Displays the Laravel version. Handy for quick version checks.--ansi
or--no-ansi
: Forces ANSI output on or off. This is about making the output colorful (or not).-n
or--no-interaction
: Skips any interactive input. Great for automated scripts.--env
: Specifies which environment configuration to use.-v
,-vv
, or-vvv
: Increases the verbosity of messages. More v’s mean more details!
Now that we’ve covered the basics, let’s dive into the specific Artisan commands. I’ll guide you through each one, explaining what they do, how to use them, and why they’re useful.
Artisan Commands: A Comprehensive Guide
App Management
about
The about
command is like asking Artisan to introduce itself and your application. It provides a quick overview of your Laravel application.
Usage:
php artisan about
Options:
--only[=ONLY]
: Displays only a specific section of the information.--json
: Outputs the information in JSON format.
This command is great when you need a quick snapshot of your application’s configuration, especially when you’re working on multiple projects.
down
The down
command puts your application into maintenance mode. This command basically puts up a “Back in a bit!” sign on your website.
Usage:
php artisan down
Options:
--redirect[=REDIRECT]
: The URL to redirect all requests to.--render[=RENDER]
: The view to be rendered while in maintenance mode.--secret[=SECRET]
: The secret phrase to bypass maintenance mode.--status[=STATUS]
: The status code to use when returning the maintenance mode response.
Example:
php artisan down --redirect=/maintenance --secret="1234"
This command is crucial when you’re deploying updates or performing maintenance on your live site.
env
The env
command displays the current framework environment. It’s a quick way to check which environment your application is running in.
Usage:
php artisan env
This command doesn’t have any unique options. It’s straightforward but very useful, especially when you’re managing multiple environments (development, staging, production).
up
The up
command brings your application out of maintenance mode. This command is how you flip the “Open” sign back on for your website.
Usage:
php artisan up
This command doesn’t have any unique options. You’d typically use this after you’ve finished your maintenance tasks and want to make your site accessible again.
Cache Management
cache:clear
The cache:clear
command does exactly what it says - it clears the application cache.
Usage:
php artisan cache:clear
Options:
--store[=STORE]
: The name of the store you want to clear.--tags[=TAGS]
: The cache tags you would like to clear.
Example:
php artisan cache:clear --store=redis --tags=users
This command is super useful when you’ve made changes to your application and want to ensure you’re not serving stale data.
cache:forget
The cache:forget
command removes a specific item from the cache.
Usage:
php artisan cache:forget <key> [<store>]
This command is handy when you need to remove a particular piece of cached data without clearing everything.
cache:table
The cache:table
command creates a migration for the cache database table.
Usage:
php artisan cache:table
You’d use this command if you’re planning to use a database for caching instead of files or Redis.
Config Management
config:cache
The config:cache
command creates a cache file for faster configuration loading.
Usage:
php artisan config:cache
This command doesn’t have any unique options. It’s incredibly useful for speeding up your application in production by combining all configuration files into a single, cached file.
config:clear
The config:clear
command removes the configuration cache file.
Usage:
php artisan config:clear
You’d typically use this command during development when you’ve made changes to your config files and want to ensure you’re not using outdated, cached configurations.
config:publish
The config:publish
command publishes configuration files from packages to your application’s config directory.
Usage:
php artisan config:publish [<name>]
Options:
--all
: Publish configuration files for all packages.--force
: Overwrite existing configuration files.
Example:
php artisan config:publish --all
This command is useful when you want to customize the configuration of a package you’ve installed.
config:show
The config:show
command displays the configuration values for a given file or key.
Usage:
php artisan config:show <config>
Example:
php artisan config:show app.name
This command is great for quickly checking configuration values, especially in different environments.
Database and Migration
db:monitor
The db:monitor
command monitors the number of connections on the specified database.
Usage:
php artisan db:monitor
Options:
--databases[=DATABASES]
: The database connections to monitor.--max[=MAX]
: The maximum number of connections before an event is dispatched.
This command is useful for keeping an eye on your database connections, especially in high-traffic applications.
db:seed
The db:seed
command seeds the database with records.
Usage:
php artisan db:seed
Options:
--class[=CLASS]
: The class name of the root seeder.--database[=DATABASE]
: The database connection to seed.--force
: Force the operation to run when in production.
Example:
php artisan db:seed --class=UsersTableSeeder
This command is essential when you want to populate your database with initial or test data.
db:show
The db:show
command displays information about the given database.
Usage:
php artisan db:show
Options:
--database[=DATABASE]
: The database connection to use.--json
: Output the database information as JSON.--counts
: Show the table row count.--views
: Show the database views.--types
: Show the user-defined types.
This command is helpful when you need a quick overview of your database structure.
db:table
The db:table
command displays information about a specific database table.
Usage:
php artisan db:table [<table>]
Options:
--database[=DATABASE]
: The database connection to use.--json
: Output the table information as JSON.
Example:
php artisan db:table users
This command is great for inspecting the structure of a particular table.
db:wipe
The db:wipe
command drops all tables, views, and types in the database.
Usage:
php artisan db:wipe
Options:
--database[=DATABASE]
: The database connection to use.--drop-views
: Drop all tables and views.--drop-types
: Drop all tables and types (Postgres only).--force
: Force the operation to run when in production.
This command is useful when you want to start with a clean slate, but be careful – it will delete all your data!
migrate
The migrate
command runs database migrations.
Usage:
php artisan migrate
Options:
--database[=DATABASE]
: The database connection to use.--force
: Force the operation to run when in production.--path[=PATH]
: The path(s) to the migrations files to be executed.--realpath
: Indicate any provided migration file paths are pre-resolved absolute paths.--schema-path[=SCHEMA-PATH]
: The path to a schema dump file.--pretend
: Dump the SQL queries that would be run.--seed
: Indicates if the seed task should be re-run.--step
: Force the migrations to be run so they can be rolled back individually.
Example:
php artisan migrate --force --seed
This command is crucial for applying database changes and is often used during deployment.
Key Generation and Encryption
key:generate
The key:generate
command sets the application key.
Usage:
php artisan key:generate
Options:
--show
: Display the key instead of modifying files.--force
: Force the operation to run when in production.
Example:
php artisan key:generate --show
This command is essential when setting up a new Laravel application. The generated key is used for all encrypted values, so it’s crucial for security.
Make Commands
Make commands are like magic spells that create new files for various Laravel components. They’re incredibly useful for quickly scaffolding your application.
make:cast
The make:cast
command creates a new custom Eloquent cast class.
Usage:
php artisan make:cast <name>
Options:
--force
: Create the class even if the cast already exists.--inbound
: Generate an inbound cast class.
Example:
php artisan make:cast JsonCast
Use this when you need to create custom casting logic for your Eloquent models.
make:channel
The make:channel
command creates a new channel class.
Usage:
php artisan make:channel <name>
Options:
--force
: Create the class even if the channel already exists.
Example:
php artisan make:channel OrderChannel
This is useful when you’re working with Laravel’s event broadcasting system.
make:command
The make:command
command creates a new Artisan command.
Usage:
php artisan make:command <name>
Options:
--command[=COMMAND]
: The terminal command that will be used to invoke the class.--force
: Create the class even if the console command already exists.--test
: Generate an accompanying PHPUnit test for the Console command.--pest
: Generate an accompanying Pest test for the Console command.
Example:
php artisan make:command SendEmails --command=emails:send
Use this when you want to create custom Artisan commands for your application.
make:component
The make:component
command creates a new view component class.
Usage:
php artisan make:component <name>
Options:
--inline
: Create a component that renders an inline view.--view
: Create an anonymous component with only a view.--force
: Create the class even if the component already exists.--test
: Generate an accompanying PHPUnit test for the Component.--pest
: Generate an accompanying Pest test for the Component.
Example:
php artisan make:component Alert --inline
This is great for creating reusable UI components in your Laravel application.
make:controller
The make:controller
command creates a new controller class.
Usage:
php artisan make:controller <name>
Options:
--api
: Exclude the create and edit methods from the controller.--type[=TYPE]
: Manually specify the controller stub file to use.--force
: Create the class even if the controller already exists.--invokable
: Generate a single method, invokable controller class.--model[=MODEL]
: Generate a resource controller for the given model.--parent[=PARENT]
: Generate a nested resource controller class.--resource
: Generate a resource controller class.--requests
: Generate FormRequest classes for store and update.--test
: Generate an accompanying PHPUnit test for the Controller.--pest
: Generate an accompanying Pest test for the Controller.
Example:
php artisan make:controller UserController --resource --model=User
Controllers are the heart of your application’s logic, so this command is used frequently in Laravel development.
make:event
The make:event
command creates a new event class.
Usage:
php artisan make:event <name>
Options:
--force
: Create the class even if the event already exists.
Example:
php artisan make:event OrderShipped
Use this when you want to create custom events in your Laravel application.
make:job
The make:job
command creates a new job class.
Usage:
php artisan make:job <name>
Options:
--force
: Create the class even if the job already exists.--sync
: Indicates that job should be synchronous.--test
: Generate an accompanying PHPUnit test for the Job.--pest
: Generate an accompanying Pest test for the Job.
Example:
php artisan make:job ProcessPodcast --sync
Jobs are great for handling time-consuming tasks in the background, improving your application’s responsiveness.
make:listener
The make:listener
command creates a new event listener class.
Usage:
php artisan make:listener <name>
Options:
--event[=EVENT]
: The event class being listened for.--force
: Create the class even if the listener already exists.--queued
: Indicates the event listener should be queued.--test
: Generate an accompanying PHPUnit test for the Listener.--pest
: Generate an accompanying Pest test for the Listener.
Example:
php artisan make:listener SendOrderConfirmation --event=OrderShipped
Listeners help you respond to events in your application, making it more modular and maintainable.
make:mail
The make:mail
command creates a new email class.
Usage:
php artisan make:mail <name>
Options:
--force
: Create the class even if the mailable already exists.--markdown[=MARKDOWN]
: Create a new Markdown template for the mailable.--view[=VIEW]
: Create a new Blade template for the mailable.--test
: Generate an accompanying PHPUnit test for the Mailable.--pest
: Generate an accompanying Pest test for the Mailable.
Example:
php artisan make:mail OrderShipped --markdown=emails.orders.shipped
This command is essential when you’re setting up email notifications in your Laravel app.
make:middleware
The make:middleware
command creates a new middleware class.
Usage:
php artisan make:middleware <name>
Options:
--test
: Generate an accompanying PHPUnit test for the Middleware.--pest
: Generate an accompanying Pest test for the Middleware.
Example:
php artisan make:middleware EnsureUserIsSubscribed
Middleware is crucial for filtering HTTP requests entering your application.
make:model
The make:model
command creates a new Eloquent model class.
Usage:
php artisan make:model <name>
Options:
-a, --all
: Generate a migration, seeder, factory, policy, resource controller, and form request classes for the model.-c, --controller
: Create a new controller for the model.-f, --factory
: Create a new factory for the model.--force
: Create the class even if the model already exists.-m, --migration
: Create a new migration file for the model.--policy
: Create a new policy for the model.-s, --seed
: Create a new seeder for the model.-p, --pivot
: Indicates if the generated model should be a custom intermediate table model.-r, --resource
: Indicates if the generated controller should be a resource controller.--api
: Indicates if the generated controller should be an API resource controller.-R, --requests
: Create new form request classes for the resource controller.--test
: Generate an accompanying PHPUnit test for the Model.--pest
: Generate an accompanying Pest test for the Model.
Example:
php artisan make:model Product -mfc
This command is a powerhouse, potentially creating multiple related files for your model in one go.
make:policy
The make:policy
command creates a new policy class.
Usage:
php artisan make:policy <name>
Options:
--model[=MODEL]
: The model that the policy applies to.--guard[=GUARD]
: The guard that the policy relies on.--force
: Create the class even if the policy already exists.
Example:
php artisan make:policy PostPolicy --model=Post
Policies are useful for organizing authorization logic around a particular model or resource.
make:provider
The make:provider
command creates a new service provider class.
Usage:
php artisan make:provider <name>
Options:
--force
: Create the class even if the provider already exists.
Example:
php artisan make:provider PaymentServiceProvider
Service providers are the central place of all Laravel application bootstrapping.
make:request
The make:request
command creates a new form request class.
Usage:
php artisan make:request <name>
Options:
--force
: Create the class even if the request already exists.
Example:
php artisan make:request StorePostRequest
Form requests are custom request classes that encapsulate their own validation and authorization logic.
make:resource
The make:resource
command creates a new resource class.
Usage:
php artisan make:resource <name>
Options:
--collection
: Create a resource collection.--force
: Create the class even if the resource already exists.
Example:
php artisan make:resource UserResource --collection
Resources are transformation layers that sit between your Eloquent models and the JSON responses that are actually returned to your application’s users.
make:rule
The make:rule
command creates a new validation rule.
Usage:
php artisan make:rule <name>
Options:
--force
: Create the class even if the rule already exists.--implicit
: Generate an implicit rule.
Example:
php artisan make:rule Uppercase
Custom validation rules allow you to extend Laravel’s validation capabilities.
make:seeder
The make:seeder
command creates a new seeder class.
Usage:
php artisan make:seeder <name>
Example:
php artisan make:seeder UsersTableSeeder
Seeders are useful for populating your database with test data.
make:test
The make:test
command creates a new test class.
Usage:
php artisan make:test <name>
Options:
--force
: Create the test even if the test already exists.--unit
: Create a unit test.--pest
: Create a Pest test.--phpunit
: Create a PHPUnit test.
Example:
php artisan make:test UserTest --unit
This command helps you create test classes for your application.
Queue Management
Queue management is a crucial part of any large-scale Laravel application. It allows you to defer time-consuming tasks, making your app more responsive. Let’s look at the Artisan commands that help you manage your queues.
queue:clear
The queue:clear
command deletes all of the jobs from the specified queue.
Usage:
php artisan queue:clear [<connection>]
Options:
--queue[=QUEUE]
: The name of the queue to clear.--force
: Force the operation to run when in production.
Example:
php artisan queue:clear redis --queue=emails
This command is useful when you need to start fresh, perhaps after deploying a major update that makes queued jobs obsolete.
queue:failed
The queue:failed
command lists all of the failed queue jobs.
Usage:
php artisan queue:failed
This command doesn’t have any unique options. It’s invaluable for diagnosing issues with your queued jobs.
queue:flush
The queue:flush
command flushes all of the failed queue jobs.
Usage:
php artisan queue:flush
Options:
--hours[=HOURS]
: The number of hours to retain failed job data.
Example:
php artisan queue:flush --hours=24
Use this when you want to clear out your failed jobs, perhaps after you’ve fixed the issue that was causing them to fail.
queue:forget
The queue:forget
command deletes a failed queue job.
Usage:
php artisan queue:forget <id>
This command is useful when you want to remove a specific failed job, perhaps one that you’ve manually resolved.
queue:listen
The queue:listen
command listens to a given queue.
Usage:
php artisan queue:listen [<connection>]
Options:
--delay[=DELAY]
: The number of seconds to delay failed jobs.--force
: Force the worker to run even in maintenance mode.--memory[=MEMORY]
: The memory limit in megabytes.--queue[=QUEUE]
: The queue to listen on.--sleep[=SLEEP]
: Number of seconds to sleep when no job is available.--timeout[=TIMEOUT]
: The number of seconds a child process can run.--tries[=TRIES]
: Number of times to attempt a job before logging it failed.
Example:
php artisan queue:listen --queue=high,low --tries=3
This command starts a long-running process that keeps checking for new jobs on the queue.
queue:restart
The queue:restart
command restarts queue worker daemons after their current job.
Usage:
php artisan queue:restart
This command is useful when you’ve deployed new code and want to ensure all workers are using the latest version.
queue:retry
The queue:retry
command retries a failed queue job.
Usage:
php artisan queue:retry <id>
Options:
--range[=RANGE]
: Range of job IDs (numeric) to be retried.
Example:
php artisan queue:retry 5
Use this when you’ve fixed an issue and want to retry failed jobs.
queue:work
The queue:work
command starts processing jobs on the queue as a daemon.
Usage:
php artisan queue:work [<connection>]
Options:
--queue[=QUEUE]
: The queue to process.--once
: Only process the next job on the queue.--stop-when-empty
: Stop when the queue is empty.--force
: Force the worker to run even in maintenance mode.--memory[=MEMORY]
: The memory limit in megabytes.--sleep[=SLEEP]
: Number of seconds to sleep when no job is available.--timeout[=TIMEOUT]
: The number of seconds a child process can run.--tries[=TRIES]
: Number of times to attempt a job before logging it failed.
Example:
php artisan queue:work redis --queue=high,low --tries=3
This command is similar to queue:listen
, but it’s more efficient as it doesn’t have to reload the framework for each job.
Route Management
Route management is a crucial aspect of Laravel development. These Artisan commands help you optimize and debug your application’s routing.
route:cache
The route:cache
command creates a route cache file for faster route registration.
Usage:
php artisan route:cache
This command doesn’t have any unique options. It’s particularly useful in production environments as it can significantly speed up route registration.
Example:
php artisan route:cache
After running this command, your routes will be loaded from a single cached file, which can improve performance. Remember to run this command after any changes to your routes!
route:clear
The route:clear
command removes the route cache file.
Usage:
php artisan route:clear
This command also doesn’t have any unique options. You’ll want to use this command during development when you’re actively changing your routes.
Example:
php artisan route:clear
Running this ensures that your application is using the most up-to-date routes, which is crucial when you’re making changes to your routing structure.
route:list
The route:list
command displays all registered routes in your application.
Usage:
php artisan route:list
Options:
--json
: Output the route list as JSON.--method[=METHOD]
: Filter the routes by method.--name[=NAME]
: Filter the routes by name.--path[=PATH]
: Filter the routes by path.--except-path[=EXCEPT-PATH]
: Do not display the routes matching the given path pattern.-r, --reverse
: Reverse the ordering of the routes.--sort[=SORT]
: The column (domain, method, uri, name, action, middleware) to sort by.
Example:
php artisan route:list --method=GET --sort=uri
This command is incredibly useful for debugging and understanding your application’s routing structure. It provides a comprehensive view of all your routes, including their HTTP methods, URIs, names, and the controller actions they map to.
Here’s a tip: I often use route:list
with grep
to quickly find specific routes. For example:
php artisan route:list | grep user
This would show all routes that have “user” in their URI, name, or controller action.
Route management commands are essential tools in your Laravel development toolkit. They help you optimize performance with route caching, ensure you’re working with the latest routes during development, and provide a clear overview of your application’s routing structure.
Certainly! Let’s dive into the Schedule Management section.
Schedule Management
Laravel’s task scheduling is a powerful feature that allows you to fluently and expressively define your command schedule within Laravel itself. These Artisan commands help you manage and interact with your scheduled tasks.
schedule:list
The schedule:list
command lists all scheduled tasks.
Usage:
php artisan schedule:list
Options:
--timezone[=TIMEZONE]
: The timezone that times should be displayed in.--next
: Sort the listed tasks by their next due date.
Example:
php artisan schedule:list --timezone=America/New_York
This command is incredibly useful for getting an overview of all your scheduled tasks. It shows you when each task is scheduled to run, making it easier to manage and debug your application’s automated processes.
schedule:run
The schedule:run
command runs the scheduled commands.
Usage:
php artisan schedule:run
This command doesn’t have any unique options. It’s typically called by your server’s task scheduler (like Cron) every minute. When executed, it determines which tasks are due and runs them.
Example:
php artisan schedule:run
While you wouldn’t typically run this manually, it can be useful for testing your scheduled tasks to ensure they’re working as expected.
schedule:work
The schedule:work
command starts the schedule worker.
Usage:
php artisan schedule:work
Options:
--run-output-file[=RUN-OUTPUT-FILE]
: The file to direct schedule:run output to.
Example:
php artisan schedule:work
This command starts a long-running process that runs in the foreground and executes your scheduled tasks at their specified times. It’s an alternative to using Cron, which can be useful in certain deployment environments or for local development.
Here’s a pro tip: When you’re developing locally and want to test your scheduled tasks, schedule:work
is your friend. Just run it in a separate terminal window, and it will execute your scheduled tasks as they come due, without needing to set up Cron on your local machine.
Storage Management
In Laravel, storage management primarily deals with file systems and how your application interacts with files. While there’s only one command in this category, it’s an important one for setting up file access in your application.
storage:link
The storage:link
command creates a symbolic link from public/storage
to storage/app/public
.
Usage:
php artisan storage:link
Options:
--relative
: Create the symbolic link using relative paths.--force
: Overwrite existing symbolic links.
Example:
php artisan storage:link --force
This command is crucial when you want to make files stored in your storage/app/public
directory accessible from the web.
Certainly! Let’s move on to the Vendor Management section.
Vendor Management
In Laravel, vendor management primarily revolves around handling third-party packages and their assets. There’s one main command in this category, but it’s a powerful one that you’ll likely use often when working with packages.
vendor:publish
The vendor:publish
command publishes any publishable assets from vendor packages.
Usage:
php artisan vendor:publish
Options:
--force
: Overwrite any existing files.--all
: Publish assets for all service providers without prompt.--provider[=PROVIDER]
: The service provider that has assets you want to publish.--tag[=TAG]
: One or many tags that have assets you want to publish.
Example:
php artisan vendor:publish --provider="Vendor\Package\ServiceProvider"
This command is crucial when working with Laravel packages. Here’s why it’s important:
-
Customization: Many packages come with configuration files, views, or other assets that you might want to customize for your application. This command allows you to publish these files to your application, where you can modify them.
-
Transparency: It gives you visibility into the default configuration and assets of a package, which can be helpful for understanding how the package works.
-
Version Control: By publishing package assets, you can include them in your version control, ensuring consistency across different environments.
Here are some common use cases:
-
Publishing configuration files:
php artisan vendor:publish --tag=config
This publishes all configuration files from all packages.
-
Publishing assets for a specific package:
php artisan vendor:publish --provider="Laravel\Sanctum\SanctumServiceProvider"
This publishes all publishable assets for the Laravel Sanctum package.
-
Publishing specific types of assets:
php artisan vendor:publish --tag=public
This publishes all assets tagged as ‘public’ from all packages.
Pro tip: When you run vendor:publish
without any options, Laravel will give you a list of all available publishable assets from your installed packages. This can be a great way to explore what’s available to publish.
Remember, after publishing assets, especially configuration files, you should review them and adjust as necessary for your application’s needs. Also, be aware that updating a package might introduce changes to these files, so you may need to manually merge updates if you’ve made custom modifications.
Certainly! Let’s wrap up our Artisan commands guide with the View Management section.
4.12 View Management
View management in Laravel involves handling the compilation and caching of Blade templates. These commands help optimize your application’s view rendering performance and clear cached views during development.
view:cache
The view:cache
command compiles all of the application’s Blade templates.
Usage:
php artisan view:cache
This command doesn’t have any unique options. Here’s why it’s useful:
-
Performance Boost: By pre-compiling all Blade templates, you can significantly reduce the time it takes to render views, especially on the first request.
-
Error Detection: It can help you catch any errors in your Blade templates during deployment, rather than when they’re first accessed by a user.
Example:
php artisan view:cache
I typically run this command as part of my deployment process. It ensures that all views are compiled and ready to go as soon as the new version of the application is live.
view:clear
The view:clear
command clears all compiled view files.
Usage:
php artisan view:clear
This command also doesn’t have any unique options. Here’s when you might use it:
-
During Development: When you’re actively changing views, clearing the view cache ensures you’re seeing the most up-to-date version of your templates.
-
Troubleshooting: If you’re experiencing unexpected behavior in your views, clearing the cache can sometimes resolve issues.
Example:
php artisan view:clear
Pro tip: I often use this command in combination with other cache-clearing commands during development. For instance:
php artisan view:clear && php artisan cache:clear && php artisan config:clear
This clears out all cached views, application cache, and configuration cache, giving me a fresh slate to work with.
Remember, while view caching can significantly improve performance in production, during development it’s often more convenient to let Laravel compile views on-demand. This way, you can see your changes immediately without having to manually clear the view cache each time.
That concludes our comprehensive guide to Artisan commands in Laravel. We’ve covered a wide range of commands, from application and database management to caching, queues, and view handling. Artisan is a powerful tool that can significantly streamline your Laravel development workflow.
Creating Custom Artisan Commands
While Laravel provides a wealth of built-in Artisan commands, one of its most powerful features is the ability to create your own custom commands. This allows you to automate tasks specific to your application.
Steps to Create a Custom Artisan Command
-
Generate the Command File
Use the
make:command
Artisan command to create a new command file:php artisan make:command SendEmails
This will create a new file in
app/Console/Commands/SendEmails.php
. -
Define the Command
Open the newly created file and you’ll see a basic structure:
class SendEmails extends Command { protected $signature = 'command:name'; protected $description = 'Command description'; public function handle() { // } }
-
Set the Signature and Description
The
$signature
property defines how your command will be called from the command line. The$description
appears when you runphp artisan list
.protected $signature = 'email:send {user}'; protected $description = 'Send emails to a user';
-
Implement the Command Logic
The
handle()
method is where you put the main logic of your command:public function handle() { $userId = $this->argument('user'); $user = User::find($userId); // Send email logic here $this->info("Email sent to {$user->email}!"); }
-
Register the Command (Optional)
Laravel will auto-discover your command, but if needed, you can manually register it in
app/Console/Kernel.php
:protected $commands = [ Commands\SendEmails::class, ];
Now you can run your custom command:
php artisan email:send 1
Advanced Features
- Arguments and Options: You can define required arguments, optional arguments, and options in your command signature.
- Prompting for Input: Use methods like
$this->ask()
,$this->secret()
, and$this->confirm()
to interactively get input. - Output Formatting: Methods like
$this->info()
,$this->error()
, and$this->table()
help format your command’s output.
Custom commands are a great way to encapsulate complex tasks or frequently used operations in your application.
Artisan Command Tips and Tricks
Aliases
You can create aliases for frequently used Artisan commands in your ~/.bash_profile
or ~/.zshrc
:
alias pa="php artisan" alias pamm="php artisan make:model" alias pamt="php artisan make:test"
Now you can use pa migrate
instead of php artisan migrate
.
Autocomplete
Laravel Artisan supports command autocompletion. To enable it, run:
php artisan completion bash > /etc/bash_completion.d/artisan
Replace bash
with your shell of choice (e.g., zsh
).
Chaining Commands
You can chain multiple Artisan commands using &&
:
php artisan migrate:fresh && php artisan db:seed && php artisan test
This will reset your database, seed it, and run your tests in one go.
Using Artisan in Code
You can call Artisan commands from within your PHP code:
Artisan::call('email:send', [ 'user' => 1, '--queue' => 'default' ]);
Maintenance Mode Shortcut
Instead of remembering the full maintenance mode commands, create a simple bash function:
function maintenance() { if [ "$1" == "on" ]; then php artisan down elif [ "$1" == "off" ]; then php artisan up else echo "Usage: maintenance [on|off]" fi }
Now you can use maintenance on
or maintenance off
.
Conclusion
Artisan is more than just a command-line tool; it’s a powerful ally in Laravel development that can significantly boost your productivity and streamline your workflow. From database migrations to job queues, from cache management to custom commands, Artisan provides a unified interface to interact with various aspects of your Laravel application.
Throughout this guide, we’ve explored a wide range of Artisan commands, diving into their usage, options, and real-world applications. We’ve seen how Artisan can help with:
- Application and configuration management
- Database operations and migrations
- Queue handling
- Cache and route optimization
- Scheduling tasks
- And much more
We’ve also learned how to extend Artisan’s capabilities by creating custom commands tailored to our specific needs.
The power of Artisan lies not just in its built-in functionality, but in its extensibility and how well it integrates with Laravel’s ecosystem. By mastering Artisan, you’re not just learning a tool, you’re embracing Laravel’s philosophy of elegant, expressive development.
As you continue your Laravel journey, I encourage you to keep exploring Artisan. Experiment with different commands, create your own, and find ways to incorporate Artisan into your development workflow. The more you use it, the more you’ll appreciate its capabilities and the efficiency it brings to your development process.
Remember, Laravel and Artisan are continually evolving. Stay curious, keep learning, and don’t hesitate to dive into the Laravel documentation or community resources to discover new features and best practices.
Did you like this article? Then, keep learning:
- Fix a common Laravel error related to transactions during development
- Learn how to fix the common 419 Page Expired error in Laravel
- Improve your database query performance with asynchronous caching
- Understand how to clear all caches in Laravel effectively
- Learn how to create a custom filesystem adapter in Laravel
- Dive deeper into Laravel's architecture best practices for 2024
- Master Laravel's maintenance mode to manage site downtime properly
- Get an easy step-by-step guide to database migrations in Laravel
- Discover how to create beautiful Artisan commands using Laravel Prompts
- Explore queue management and troubleshooting in Laravel applications
4 comments
Nice article, just one missing step before running the migration.
You should run the following command to generate the migration files
php artisan vendor:publish –provider=“Laravel\Pulse\PulseServiceProvider”
Thanks a lot, I just updated the article!
Hello Benjamin, Very nice article. I have installed Laravel Pulse today, and when i try to access to the url i get the next error “This page has expired. Would you like to refresh the page?” and i cannot close. Can you help me please?
Hello Joan. Did you figure this out already?