Laravel Dropbox Driver package: how to install and use it
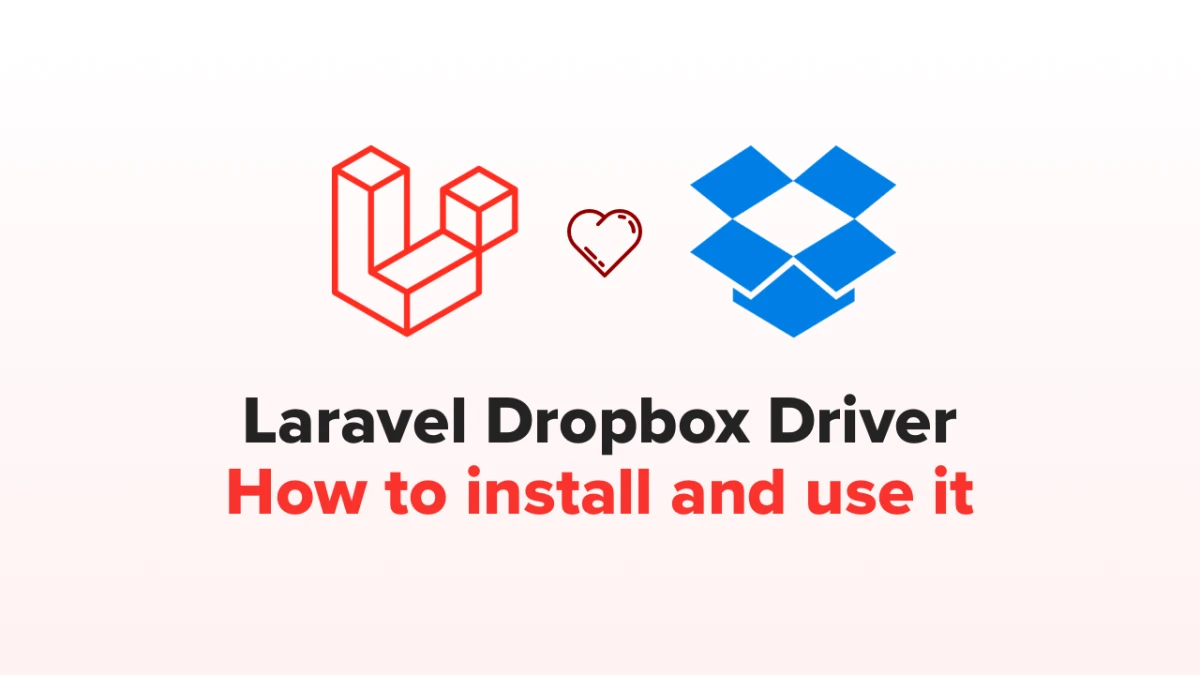
Adding a new disk in the storage is easy. The only things I did was:
- Copy and paste code from the documentation and made it a package (https://laravel.com/docs/filesystem#custom-filesystems)
- Use the Flysystem adapter from Spatie, which Laravel is based on (https://github.com/spatie/flysystem-dropbox)
Requirements
Laravel Dropbox Driver requires:
- PHP 8.1+
- Laravel 9+
Installation
To install Laravel Dropbox Driver, run the command below:
composer require benjamincrozat/laravel-dropbox-driver
Usage in your project
Add the following in app/filesystems.php:
'disks' => [ 'dropbox' => [ 'driver' => 'dropbox', 'token' => env('DROPBOX_TOKEN'), ], ],
Then, in your .env file:
DROPBOX_TOKEN=your_access_token
Get a token from Dropbox
Log in to your Dropbox account and create a new application to generate your access token.
https://www.dropbox.com/developers/apps/create
License
Take this package and do whatever the f you want with it. That’s basically what the WTFPL license says.